JavaScript at command line with the V8 compiler
A JIT compiler that can run in a browser or standalone to use JavaScript locally for scripting.
This compiler is provided as open source by Google which allowed the development of tools such as the application server platform Node.js.
Its speed of execution in effect allows the use of JavaScript to make software systems. It does not interpret the code but compiles it before you run it, although the variables are dynamic and therefore may change type during the execution of the program. But techniques are available as type inference to handle this problem.
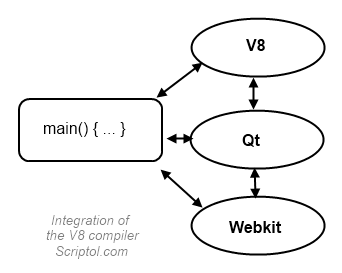
V8 has a garbage collector (stop-the-world, it stops the execution for a cleaning cycle), an advantage over the C++ language. It uses an assembler based on that of Smalltalk (Strongtalk), to create native code from high-level bytecode.
It implements (in 2012) ECMA-262, the third edition of JavaScript, and works on Windows, MacOS and Linux (ARM or x386).
V8 is very quick and we wonder if it needs for Asm.js at the sight of the last benchmark (October 2013) of the Google team, which compares the V8 compiler with the compiler of Firefox, both with Asm.js code.
Comparative performance of Asm.js code on Firefox and Chrome (Source Google).
A value of 1 means once the speed of the binary code.
Some thought Asm.js had been implemented in Chrome. This is not the case, the speed of V8 is such that in some cases, the JavaScript code (and not only the Asm.js subset), runs as fast as with the specialized compiler Asm.js of Firefox!
Using V8 as a scripting tool
JavaScript may therefore be used as scripting language with V8 and possibly the scripts built with GUI by integrating them into C++ code and graphics libraries.
V8 is designed as a library that can bind to any C++ project and then the compiler used as a function of a larger system.
This is what the Chrome browser does, for which it was made, and to which it gives its top speed of execution compared to competitors. But they tend to adopt the same techniques and catch up.
It can be embedded in a C++ program as a library or be used standalone, as does an interpreter, to parse a JavaScript program. In the second case we need to create a main function that is used to call the parser, provide a source code to compile and display the result of the compilation.
But the generated code can interoperate with libraries included in the project.
In business terms, to run a program, you must create a C++ launcher that we will bind to the V8 code before compiling it. This creates a parser and interpreter that can execute JavaScript.
int main(int argc, char* argv[])
{
String source = String::New($argv[1]);
Script script = Script::Compile(source);
Value result = script->Run();
String::AsciiValue ascii(result);
printf("%s\n", *ascii);
return 0;
}
The detailed version and instructions for building the JIT interpreter can be found on the V8 site.
But there is a much simpler solution.
Running JavaScript code at command line
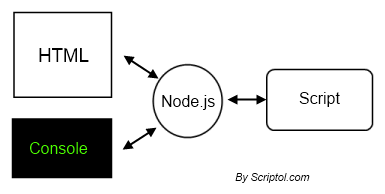
Node.js is a server library written in JavaScript, intended for applications that can grow without limit over time. It is asynchronous and reacts like HTML applications to events.
It is used by Microsoft for the Azure service, by eBay, LinkedIn for mobiles, the Yandex search engine, by Yahoo for the Manhattan project.
And you can use it you too to run your own scripts.
Suppose your script is in the file hello.js.
Download and install Node.js, you enter the directory where is the node.exe executable, and type:
node hello.js
That's all!
JavaScript IO at command line
To communicate with the script, to provide it information, Node has the process object.
The process.argv attribute contains the parameters passed on the command line.
Thus if the command is node hello.js me, the contents of process.argv will be:
- node.exe
- hello.js
- me
The length attribute specifies the number of arguments:
process.argv.length
And to show results, you may use the console object.
console.log("Hello you!");
The complete demo script:
console.log("Arguments:" +process.argv.length);
var name = process.argv[2];
console.log("Hello " + name + "!");
But using Node.js, we can go far beyond that ...
- Access the file system.
- Uses a HTML 5 interface with WebSocket.
- Create a local server ...
- etc...
Demonstrations are given in this JavaScript folder.
More implementations to use JavaScript from the command line
- Narwhal. Designed to use different JavaScript interpreters.
- K8. Adds input-output functions. (Linux).
Reference