AspectJ, Aspect Oriented Programming in Java
Aspect J is a Java extension that implements Aspect-Oriented Programming (AOP), a technique that modularize crosscutting concerns, ie can apply the same process to different programs.
AspectJ was made
available in 2001 and AOP is developed at Palo
Alto Research Center since 1994.
Here the unit is not the class, but
a concern, that spans multiple classes. Concern may be for example, properties,
area of interest of a system and AOP describes their relationship,
and compose them together into a program. Aspects encapsulates behavior
that concern multiple classes.
Aspects of a system can be inserted, changed, removed at compile time.
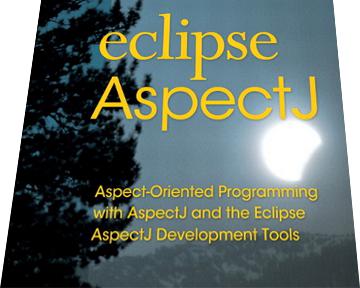
The difference between an aspect and a class can be expressed as an example. Suppose management user registration is a class, then it is composed of methods used by each program that uses the package. But if user registration is a "concern", then it mixes with other packages of each program using it. As a class, it may be replaced by a different concern. The concern provides interaction with other processes while the class knows only its own attributes.
Using AspectJ can reduce dramatically the size of a program without a lost in performance, and simplify the design in the same degree. It improves modularity and reusability of code. And it is especially useful for debugging large projects.
Adding AOP to Java
Providing aspects to Java code is based on the following principles:
- Add-on to Java: may be added to an existing program (and replace lot of code or add some controls or debug processings to it).
- Join points represent defined point in a program's execution as method call, exception catch, and so on.
- A join point may contain other join points.
- Pointcut is a construct that picks out a set of join point,
according to defined criteria.
For example, the set of all methods execution in a package. - Advice is a code that executes before, after or around a designed join point.
- The ajc compiler generates from AspectJ source either .java sources or directly .class bytecode files.
- Aspect code may be reused throught programs.
Sample code
This aspect concerns the execution of any method in
the Eclipse program.
An advice executes some code before each method is called, and an other
one executes some code after the call.
public aspect xxxx { pointcut anyMethod() : execution (* org.eclipse.. *(..)); before(): anyMethod() { ... some code... } after() : anyMethod() { ... some code... } }
Tools and documentation
- Eclipse AspectJ
The AspectJ project as part of Eclipse. - DeveloperWorks
Read the article of N. Lesiecki.
Updated on July 2014.