PHP, a language for building Web pages on the server
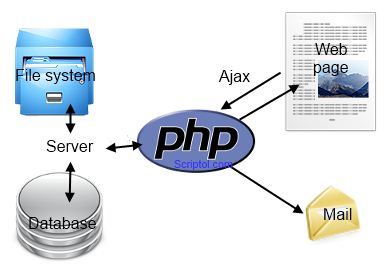
There are different views on how to optimize language to speed up its operation, and Facebook has even taken a radical approach by creating a compiler and a virtual machine. But this language became universal on the server side. PHP is the P of LAMP, a popular architecture that includes the Linux operating system, the Apache server and the MySQL database manager.
A PHP script produces web pages, and may be embedded inside HTML code as JavaScript but works server side. The interpreter may be used locally on the desktop.
The language was designed in 1993 by Rasmus Lerdorf because it needs a free tools to program Web pages and it was released under the name PHP/FI, Personal Home Pages / Form Interpreter.
"I never thought while writing PHP that someday millions of people will be looking over my shoulder into my code. If I would have known that, I would have surely done a few things differently".
Rasmus Lerdorf, 2012.
Having worked at Yahoo!, Rasmus Lerdorf then was employed at WePay and then Etsy. He then developped PHP further with the help of other programmers and made
it open source for the community of users. A new engine was created for
PHP 3 and a new name given: PHP Hypertext Preprocessor in 1997. Recursive acronyms were the trend with name like: GNU is Not Unix, PNG is Not Gif and so on...
The Zend
engine was created in 1999 for PHP 4.
PHP 5 was released in 2004, it is more object-oriented and supports XML. Execution speed was doubled going from PHP 5.3 to PHP 5.6.
PHP 7 was released on December 3, 2015. There are no PHP 6 after the project aborted some of its features were transferred to PHP 5.
Features of PHP
PHP borrows ideas from C and the shell of Unix.
- The syntax of C, minus typed variables, plus:
- Dynamic untyped variables are prefixed by $.
- Object oriented.
- Associatives arrays (tables with keys).
- A foreach construct to scan arrays and iterators.
- String interpolation: you can place variables in strings.
- Offers a lot of APIs dedicated to the standard of the web and databases.
Syntax
- The language is not case-sensitive.
- Floating point numbers are denoted by a dot followed by a number or zéro.
- Variables are prefixed with a $ symbol and no type is specified in advance.
- Literal string which use the "" notation are evaluated for variables and special chars, between ' ' they are not.
- <?php and ?> must enclose a PHP program.
- # or // starts a comment.
- array( "1" =>" "a, ...) is a dictionary.
- const is introduced in PHP 5.6.
Control structures
The if structure has elsif and else options.
if(x < 10) {
echo "$x less than 10\n";
}
elseif(x > 10) {
echo "$x greater than 10\n";
}
else {
echo 'etc...\n'
}
The while structure:
while(expr) {
...
}
Function or method
The definition of a function starts with the function keyword, followed by the
name and the list or parameters separated by commas, and the body is enclosed
between { and }.
The return keyword in the body of the definition allows to return
one value.
function funcname( arguments ) {
...statements...
return(x);
}
Class
class name {
...
}
The body is similar to the global code.
String
Example, displaying the content.
$str = "demo";
$len = strlen($str);
for($i = 0; $i < $len; $i++) {
echo $str[$i];
}
Array
Declare, merge two arrays, extracting a subset, displaying the items.
$arr = array(1,2,3 );
$arr = array_merge($arr, array( 4,5));
$sub = array_slice(|$arr, 1,3);
foreach($sub $as $num) {
echo $num;
}
Should display 234.
Built-in local server
To work locally, including for development, you had to install a server and then run the script in a browser with the prefix http://localhost.
Since version 5.4, it becomes redundant as a local server is included in the distribution. The server is started with this command:
php -S localhost:1100
Where 1100 is the port number (this is an example). To launch the index.php script, and display the result in a browser, type in the URL field:
localhost:1100
The advantage over solutions like Wamp is that it is not necessary to move the files to a specific subdirectory inside the directory of Wamp, www in this case. Scripts and PHP pages are working where they are.
Why use PHP? The user experience
PHP is an Internet tool, running server side,
it is a scripting language that may be embedded into web pages. It is
usable for processing large data and build a HTML page that display the
results. (JavaScript is convenient for dynamic change of HTML pages.)
PHP 5 is a simpler concurrent to Java as an application server and a platform
for web applications and web services. It eases the use of XML, MySQL,
SQLite, SOAP and many database systems.
It is the most used language to build a CMS, a content management system.
The language was developed from day to day, to add the features needed, without well-defined plan. As a result it lacks consistency, clear rules, and we must constantly refer to the manual, especially to find the parameters of a particular function.
For example arguments for the substr_replace function are the original string followed by the part to be replaced and the replacement string, while the str_replace function has for arguments the part to be replaced and the replacement string followed by the original string.
The fact that an array is also a dictionary, so that the indices are keys, is a source of headaches for programmers.
Read more about PHP inconsistency in PHP, a fractal of bad design. But this article is not without errors. For example, PHP has a debugger (see the list of tools below), it works without Apache, constants are not functions, etc...
There are actually many alternatives, Python, Ruby, Node, Go, and more recently, Julia.
PHP 6 (actually PHP 5.x)
The PHP 6 project with Unicode support has been abandoned, but some features seemed so useful that it was decided to incorporate them into PHP 5:
- Namespaces.
- Generators and coroutines (PHP 5.5).
- XMLReader. To read XML files in Sax mode.
- XMLWriter.
- goto, this directive comes from the Basic language.
- Closures.
- Filefinfo eExtension, to access the file system.
- Phar. Allows to embedd a PHP application into a single executable file, as does Java with .jar files.
- Intl. Internationalization.
- The operators continue and break will work with a constant parameter or without parameter.
- The ereg() function for regular expressions will be deleted. It will be replaced by preg_match() whose format is different but more generally used.
- The compatibility with older formats like GD 1 and Freetype 1 will no longer be supported.
- Magic_quotes, that was very decried as a source of security holes will no longer be supported.
- The same is true for register_global, safe_mode and register_long_arrays.
We must therefore modify the current applications to make them compatible. - The tags <% %> will no longer be recognized, if you use <?php ?>, there will be no change.
- dl() to load dynamically a library is disabled since 5.3.
- Array_column returns a column from a multi-dim array.
Support for Windows XP is dropped since 5.5.
In 2008, Zend CEO, said in an interview:
So we are anticipating a long rollout cycle for PHP 6, and we did not want to take the same route that the Perl project did, with project contributors still working on Perl 6 I think six years later. People make fun of Microsoft, but take a look at Perl 6...
In 2015, PHP 6 still does not exist, and they preferred to skip it to PHP 7. Actually, Perl 6 is still not finished either!
PHP 7
Formerly called PHP Next Generation (PHPNG), this new version succeeds to PHP 5 (there will never be a PHP 6), and wants one hand faster performance with a JIT interpreter lake HHVM and on the other by making the syntax more consistency. The performance gain is 100% on average compared to 5.6.
Asynchronous programming make parallel processing possible.
- The language supports types for the arguments and return of a function.
function mult(float $a, float $b) : float { return $a * $b; }
- A new operator (Spaceship operator) is added; <=>. It returns 0 in case of equality of the two compared values 1 if the first is greater than the second, and -1 in the opposite case.
- Generator, with the yield keyword.
- Constant array, sort of indexed enum.
- Closures (see dictionary).
- Filteted unserialize() function for security.
- Unicode.
- A set of new reserved words is added: bool, int, float, string, numeric, NULL, TRUE, FALSE, resource, object, mixed.
- The list() structure does not always follow the same order to assign variables.
Should we move a site to PHP 7? This allows better performance and memory saving, so it is certainly recommended. However, we must ensure that there is no incompatibility issues as a set of obsolete but still active functions is now removed. For example, the mysql functions.
Optimizing PHP: Google vs. PHP Team
Technicians at Google advise Webmasters how to optimize the PHP code and indicates several points on how to change the code, replace control structures, to optimize it at best.
But the creators of the language have a different analysis. This controversy is interesting for webmasters that use PHP on their site because it provides useful information on optimizing the code.
- Copying variables or not?
In fact PHP optimizes the allocation and value of physical variables is copied only when it changes. - Single or double quotes?
This makes no difference. Although there are variables to interpret inside the strings between double quotes, it is not slower than concatenating variables and pieces of strings. - Echo or print?
The execution speed is similar, but it some cases, this may depend on the configuration of the server.
(The question of speed, is useful when generating HTML pages from PHP commands.) - Switch/case or if/else?
Again, using one or the other makes no difference because the internal code is the same.
The only point on which both parties agree, is that it is best to migrate to the latest version of PHP , as it is always the fastest.
HHVM
HHVM (Hip Hop Virtual Machine) is a JIT compiler created and used by Facebook, available on GitHub with the source code. Running on the HHVM virtual machine, PHP is twice faster than on the Zend interpreter.
HHVM replaced PHP 5.3 at Wikipedia too (they plan to move to PHP 7 now).
Comparisons with PHP 7 showed that HHVM is slightly faster for the legacy code, but when the code takes advantage of the new features of the language, it's the PHP 7 interpreter the faster.
For an analysis of its operation, a detailed article: LLVM code generation in HHVM. LLVM is now the final backend in a chain of intermediate representations
.
PHP is firstly compiled to HHVM bytecode, then in a second intermediate representation. It is then translated into assembly language which can be converted into LLVM bitcode.
The virtual machine works with the Hack language that replaced PHP and became incompatible with it in 2018. It may be replaced by PHP 7 without loss in performance.
See also the history of HipHop.
Tools and tutorials
PHP Editors
Most editors have syntax-hightlighting and auto correction for PHP, Visual Studio Code for example. IDEs could add more tools.
- NetBeans
IDE from Sun written in Java for PHP and other languages.
NetBeans takes advantage of the built-in PHP server, provided you configure the "Run application" property of the project to do so. When you launch the current script from within the editor, you see the results displayed in the default browser. - Aptana Studio
Includes a PHP editor. (Windows) - Eclipse IDE
Extension to Eclipse for a PHP development interface.
Development tools
- Php.net
The official website, home of the interpreter and the language. - Hack.
Statically typed version of PHP, compatible with the mainstream language until 2018.
- HippyVM
Another virtual machine, faster and that puts a bridge with Python. - XAMPP Server
Windows Apache Linux PHP local server. Put your PHP script in the htdocs sub-directory or www at root of the Xampp/Wamp directory and they can run as on a true server, with MySQL requests and PhpMyAdmin or SQLite Manager to manage the databases! - Xdebug
Interactive debugger for PHP.
Scripts and tutorials
- SQL tutorial
with PHP and MySQL
Learn with examples to make PHP scripts for using SQL on a website. - JavaScript in PHP. How to integrate the V8 compiler into the PHP language.
- PHP reboot. Proposal for a reboot of PHP without $ and other antiquities. This is actually the Go language.
See also : PHP frameworks.