C++, a system language and for fast applications
The C language has been augmented with objects to facilitate the representation of entities to be processed, and code reuse. This has transformed this system language created in 1972, into an higher level language, C++.
Other features have been added over time as templates, tuples, concurency.
C++ was created in 1981 by Bjarne Stroutstrup, who discovered the object orientation in Simula 67 and wanted to add it to the C language while remaining compatible with it and thus preserving its advantages, including portability between systems.
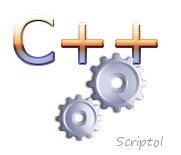
ts design follows a set of rules:
- It is defined according to actual problems and its features address them immediately.
- It is more important to provide features that to prevent misuse of language.
- Types created by the user are as efficient as predefined types.
- Performance is not penalized by unnecessary functions.
- There should not be any intermediate language between C++ and the native code.
The first ISO standard for the C++ language date of 1998, it is C++ 98. A new version was created in 2011, C++11. With this version that brings nothing to readability and modernity, the language is rooted in its role as a system tool that has been diverted for a time.
The increasing complexity of C++ in version 11, 14, etc... was criticized especially by Donald Knuth and Edsger Dijkstra. The designer himself was not happy with all the features added yo the syntax within time and he is has once referred to the history of the Vasa, a Swedish boat that has been added so much that it sank after the very first meters. Bjarne Stroustrup:
Within C++, there is a much smaller and cleaner language struggling to get out.
It is used as application language thanks to graphical interface such as Qt and GTK and with 3D engine such as Unreal Engine. It is clear that it was designed to optimize performances of the computer and certainly not the programmer productivity. Errors that inevitably come with its hard-core and unreadable syntax are paid dearly in time debugging. Used where appropriate, for system tools, it provides undeniable advantages over C, such as RAII, which will be discussed below.
Sample of code: Merging and displaying strings.
string s = "demo" + "trail";
int l = s.length();
for(int i = 0; i < l; i++) {
char c = s[i];
printf("%c\n", c);
}
Short history of the language
- In 1979, Bjarne Stroutstrup was working on a Ph. D. thesis in computer science and discovers Simula 67.
- He began to work on a language called C with Classes. The goal is to keep the speed and portability of C and adding object orientation.
- In 1981, he add to C: classes, inheritance, inline functions, default argument of functions. Its compiler is called CFront but merely translates the code in C.
- In 1983 the language was renamed C++. Support is added to virtual methods, method overloading, references with &, constant methods, the symbol // to one-line comment.
- In 1985, the first book to describe the C++ programming language is published: "The C++ Programming Language".
- In 1989, were added static and protected members, multiple inheritance.
- In 1990 is released the Turbo C++ compiler (from Borland) along with essential libraries.
- In 1998 is defined the first ISO standard.
- Then are added templates, exceptions, namespaces, the bool type.
- In 2003 the standard was revised.
- In 2011, after six years of work and delays, appears a new standard. Are added a for in loop, comcurrency, dynamic variables with the auto keyword.
- In 2014 is planned a new ISO standard.
Objects of activities formalized
C++ describes classes into header files, and body of methods into
source files. By declaring instances of classes you can reuses sets
of variables and methods without to define them again.
Overloading allows to redeclare a method with different parameters but the same name.
Classes inherits one from other and share their methods. Multi-inheritance remains specific to C++, this has not been reused on derived languages.
Over time, the definition of struct has evolved to become equivalent to that of a class, with the difference that a structure is public by default when a class is private by default. Structs can have methods and support inheritance and in fact we can avoid the use of classes entirely in an OO program.
This conceptual model is criticized by Trygve Reenskaug, inventor of the MVC and DCI, for who it is too static and poorly adapted to the reality of processing in which the representation of objects should vary depending on the context. But this drawback is shared by all current object oriented languages except perhaps by those which like Go where inheritance is replaced by composition.
Managed memory
C++ introduced RAII (Resource Acquisition Is Initialization), the principle that when an object is created and thus a memory area is required, it will be released automatically when the object is destroyed. This avoid the need of a garbage collector and of the free command like in the C language.
The resource can be an object, a class, an instance of file. It is declared in a scope and released when leaving the scope.
C++11
The new ISO standard of the C++ language is extended, said Bjarne Stroustrup, its creator, according to three levels:
- Language.
- Standard library.
- Concurrency.
The definition of the standard has been approved by the ISO C++ comittee on March 25, 2011.
The internal UTF-8 format is now supported for strings.
New types and keywords
- auto
Type of variable deduced from what is assigned. Example:vector <string> v = ( "Alicia", "Bea", "Clara", "Dara"); for (auto x: v) cout "x" '\ n';
- constexpr
A constant expression. - nullptr
A reserved word for a null pointer.
Ex:char *x = nullptr;
- raw string
String where escape codes are not interpreted, as in PHP with single quotes.
They have the R prefix. - decltype
How to use the type of an expression, returns the type and modifiers of any object including a function. - UTF 8 string literal
u8"Some text".
u is used for UTF 16 and U for UTF 32.
Security on types
- Control of alignment of values.
- Control of default values.
New constructs
For loop over an interval.
As it is implemented on most scripting languages.
int arr[5] = {2, 8, 21, 56, 995};
for (int &x : arr) {
printf("%d\n", x);
}
As you can see, the language retains the principle of minimal source code. It does not use the keyword in as do most languages, but a symbol instead.
A tradition that was born when it was necessary to save each character to reduce the size of code! Memory was expensive in 1972 ...
Lambda function.
Functions defined in place for a contextual use.
rvalue.
This is a
reference to the contents of a variable, where the A variable is assigned the content of B
without a copy but by moving the pointer on the new content, in the
perspective where B is no longer used.
Variadic template.
Template whose number of arguments is variable.
Static assertion.
Introduced with the keyword static_assert, it tests the validity of an assertion at compile-time.
Typed enum with scope.
Classes
Delegating and inheriting constructors. The constructor of a class can call constructors of other classes.
Attributes of classes may be assigned directly in the definition of the class.
Standard library
New object appear that already became familiar in scripting languages such as PHP.
- regexp
Regular Expressions. - tuple
Used in the Python language. - Array
Static array. - unordered_map
Hash table. - Date.
- Smart pointer.
And many various components of libraries and improvements. For example, now you can assign a static list to a vector at its declaration.
Concurrency
Multiple threads with shared memory are easy to use. To make it, we create a function and call it through a function pointer in the thread command ...
#include <thread>
void f(int a) {
... code...
}
int main() {
...
thread t(&f, 1000);
t.join();
...
}
The join() command starts the thread.
he async command calls a function asynchronously, something that has become familiar with Ajax.
Features for concurrency in C++11:
- Memory model adapted to today's computers with multi-core processors.
- Threading ABI.
- Atomic types.
- Mutexes and locks.
- Thread local storage.
- Exchanging messages asynchronously.
Security
C++ is a language that gives full freedom to programmers, but it is not a secure language. The NSA (National Security Agency) in the USA does not recommend its use due to possible vulnerabilities in memory usage. The miscreants could use the loopholes that appear with a flexible language. When security is essential, organizations should instead use other languages such as C#, Go, Java, Swift or even Rust for the masochists.
Tools and documents
- No Make builds a C++ project with no makefile.
- Visual C++. Visual Studio Community by Microsoft. Free IDE for C++ developement with ou without .NET.
- Qt Creator.
Cross-platform IDE based on the Qt framework. A graphical user interface designer is embedded (click on .ui files).
- Eclipse. IDE and tool integrator with a plugin for C++. (Java)
- MingW. This is the Windows version of GCC, the free compiler from the Free Software Fondation.
- CLang. Fast C, C++ and Objective C compiler, frontend to LLVM that produces portable intermediate code, which could be also converted to binary or JavaScript.
- Cling. Interpreter for C++, using a JIT. Very useful tool to learn the language or to make scripts.
- C to C++. Converts a C project to C++. (Python 2)
- Google C++ style guide. Use a clean subset of C++ for security and readability.
- Boost. Open source libraries for C++.
Objective C is another object oriented version of the C language, simpler than C++. GnuStep is a objective-C IDE for Linux and Windows.
See also: The candidates successor to C++.