Drawing an ellipse with the Canvas' arc method
Like for drawing a circle, we use the arc method but the radius change depending on the direction.
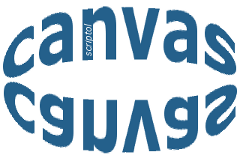
In fact we are going to draw an ellipse, which is a more precise case of an oval. According to Wolfram, the oval does not have a mathematical definition, unlike the ellipse (although all kinds of ovals correspond to a formula). The ellipse is like a circle seen in perspective. It is defined by a geometric figure with two axes of symmetry, while the circle is entirely symmetrical.
So, for simplicity, say that what we draw here is an oval with double symmetry or a circle flattened!
Drawing an ellipse is done with the scale method. This method modifies the proportions of an object after it is defined.
scale(x, y)
The arguments are the values of horizontal and vertical ratio. If we give such value to x as 0.5, the image will be reduced by 50% in the width direction.
If you want to create an ellipse that is twice as wide as high, we give the value 1 to x and to y the ratio of 0.5.
<canvas id="canvas3" width="400" height="200"></canvas>
function oval()
{
var canvas = document.getElementById("canvas3");
var context = canvas.getContext("2d");
context.lineWidth="4";
context.strokeStyle="green";
context.scale(1, 0.5);
context.beginPath();
context.arc(200, 200, 180, 0, 2 * Math.PI);
context.stroke();
}
oval();
We must point out one thing. Although the height is 100 pixel canvas, it gave the arc method a value of 100 for the argument y.
This is because the scale method are applied to the coordinates of the figure inside the canvas and not just its shape.
The ratio of 50% of the argument y of scale applies to the argument y of arc too, so the the vertical position of the shape.
Unfortunately the ratio also applies to the width of the line, so that the ellipse has not the even thickness elsewhere.
Full code for drawing a regular ellipse
To have a regular line, we must restore the initial context after the invocation of scale and before that of stroke, so that the modification of the proportions is canceled with regard to the thickness of the line (while still applying to its shape).
The final code for drawing any oval shape:
<canvas id="canvas4" width="400" height="200"></canvas>
function drawOval(x, y, rw, rh)
{
var canvas = document.getElementById("canvas4");
var context = canvas.getContext("2d");
context.save();
context.scale(1, rh/rw);
context.beginPath();
context.arc(x, y, rw, 0, 2 * Math.PI);
context.restore();
context.lineWidth=4;
context.strokeStyle="orange";
context.stroke();
}
drawOval(200,200,180, 90);
Parameters of the drawOval function are x and y, the center of the shape, and the horizontal and vertical radius.
The ratio method for the vertical scale is then the ratio between these two radii, rh / rw.
Documents and related