Drawing speech bubbles in Canvas
How to make a comic bubble and put a quote inside.
If Canvas is not supported by the browser, the text is just displayed without the drawing of the speech bubble.
Demonstration
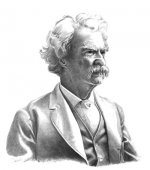
Marc Twain.
HTML code
<div class="bubble">
<canvas id="canvas1" class="bubbleborder" width="240" height="200" >
</canvas>
<div class="bubbletext">
All generalizations are false, including this one. <br><i>Marc Twain.</i>
</div>
</div>
Two layers are absolutely positioned in another, both with top = 0 left = 0, so that they overlap.
One is a canvas used to dessign the bubble.
The other is a classic <div> used to display the text. To center the text in the bubble, the padding property is applied to the layer that contains it.
It is not possible to format text directly in canvas.
JavaScript code
function drawBubble(ctx, x, y, w, h, radius)
{
var r = x + w;
var b = y + h;
ctx.beginPath();
ctx.strokeStyle="black";
ctx.lineWidth="2";
ctx.moveTo(x+radius, y);
ctx.lineTo(x+radius/2, y-10);
ctx.lineTo(x+radius * 2, y);
ctx.lineTo(r-radius, y);
ctx.quadraticCurveTo(r, y, r, y+radius);
ctx.lineTo(r, y+h-radius);
ctx.quadraticCurveTo(r, b, r-radius, b);
ctx.lineTo(x+radius, b);
ctx.quadraticCurveTo(x, b, x, b-radius);
ctx.lineTo(x, y+radius);
ctx.quadraticCurveTo(x, y, x+radius, y);
ctx.stroke();
}
function dispQuote()
{
var canvas = document.getElementById('canvas1');
var ctx = canvas.getContext('2d');
drawBubble(ctx, 10,60,220, 90, 20);
}
window.onload=dispQuote;
The text features of Canvas are not used, they are useless here.
CSS code
.bubble
{
position:relative;
background:green;
float:left; /* because the image at left */
}
.bubbleborder
{
position:absolute;
top:0;
left:0;
}
.bubbletext
{
position:absolute;
top:0;
left:0;
width:220px;
height:200px;
padding:76px 8px 8px 8px;
text-align:center;
}
How to generalize the script
All generalizations are false, but it may however be useful to generalize the script for texts of different lengths.
This can be achieved by adjusting the parameters of the bubble in the JavaScript code to the length of the text.
- The JavaScript String.length function returns the number of characters.
- Depending on the font family and font size you choosen, are determined the values w (width) and h (height) of the bubble that are the 4th and 5th arguments of the function drawBubble .
Back to Canvas.