Dart, an alternative to Java for smartphone applications
Dart allows you to create cross-platform applications with the syntax of a very classic language.
This language from Google is available at dart.dev. It may be used on Android to replace Java or on iOS.
It implements concurrency in the form of communicating actors with their own environment and has true classes.
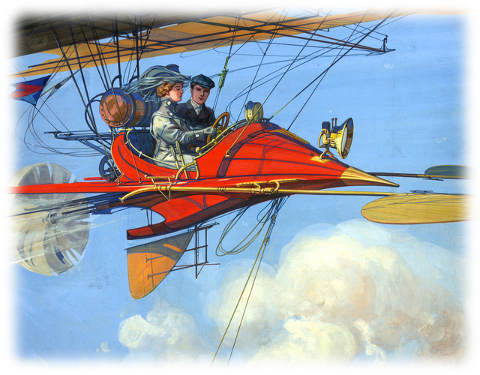
The future is never what it was
Faced with fundamental design problems in JavaScript that it see not solved by incremental improvements, Google opted for a radical solution: completely replaces the language with another, with a syntax that suppresses all the drawbacks of JS. But JavaScript is a language that is interesting in its overall design, it is the details that are weak and Dart unfortunately change also this innovative design to return to the classics.
On the browser, there is a better solution: to allow using any programming language in frontend, to a bytecode, a role now fulfilled by WebAssembly.
Google promises that programming in Dart will become interactive thanks to tools, you will edit and run a program directly, and change the code according to the results. For a Web application, it will be a big advantage.
Programs may be evolving. You can begin with a simple script with dynamic variables, which can be transformed into application where you add typed variables and classes.
Dart was competing with SoundScript (from Google too) a JavaScript extension that brings typed variables and other features that increase performances, while suppressing JS drawbacks.
Google announced that it would not implement the Dart VM in Chrome browser in 2015. But Dart may still be compiled to JavaScript like TypeScript. Google uses Angular 2 Dart for some of these internal projects although Angular by default works on TypeScript.
Dart is a langage to build mobile applications for Android and iOS whit the Flutter interface and is also the programming language of Andromeda (or Fuchsia), a future Google OS designed for security, with Flutter too. We will follow the developments of this project.
A modern version of C with classes
The syntax of Dart, created in 2011, is more than classic, it's actually near that of C (1972) and JavaScript (1995). t can be defined as a simplified version of C++.
A Dart program can be executed by a virtual machine for a desktop or mobile app (Dart Native), or compiled into JavaScript and thus produce a code usable by all browsers (Dart Web).
- The main function executes the code after loading, and not step by step while the page is parsed as JavaScript.
- Classes and interfaces.
Unlike JavaScript (before ECMAScript 6), in Dart you can declare classes rather than dynamic objects, and have single inheritance. It does not allow overloading methods. - Fonctions.
A function is expressed as in C (and not with the function keyword as JavaScript), except that the return type is optional. If not specified, it is dynamic.
As in JavaScript, a function may be embedded into another function. - Optional types.
The programmer can use dynamic variables to a script or typed variable for security on a larger project or to speed up the processing. - Asynchronous. We may launch asynchronous operations with await or promise, called here Future.
- Concurrent.
Entities named Isolates can operate concurrently. They communicate by messages but have their own memory space.
So the language is not multi-threaded, but has parallel processes, sort of actors. - The + symbol is used for the addition or concatenation. It can also be overridden by class methods.
- Self-documentation easier.
- Like in Scriptol, a name should not be declared locally within a block when it already exists in a containing block (here you get a warning, in Scriptol this a syntax error).
- An object is public (used outside the library), or private, in this case it is prefixed by an underscore.
- Classes can include getters and setters. The syntax is:
type name => expression. - A method can be static. It is then declared with the static prefix. The same applies to attributes.
- Operators are those of JavaScript. This includes === and !== for strict comparisons.
- As in PHP you can insert variables in strings with the $ prefix.
- Reserved words:
abstract, asset, async, await, wagon, box, catch, class, continue, do, except, extends, factory, false, finally, for, Future, get, if, implements, import, interface, in, include, is, library, negate, operator, proxy, set, source, static, switch, throw, try, true, typedef, until, while. - List of types and builtin objects:
bool, Date, Double, Duration, int, list, map, number, Isolate, Object, Match, Math, Pattern, Queue, RegExp, String, var, void. - Mixin
A class that can be included in another to form a composite object. Unlike inheritance that is simple here and not multiple, we can incorporate several mixins (see dictionary) in a class. - Snapshot.
A snapshot is a form of persistence of the state of a program. Its data are stored on disk for use at the next session. This allows you to instantly restart a program as if it was in standby. (The current documentation does not mention it but the snapshot code is part of the source of the virtual machine). - Streams.
Functional reactive programming is added through streams, that updates automatically a variable declared as dependent of values from other variables and events. - SIMD.
Instructions operating in parallel. - async and await. (1.9).
Allows in a function to process sequentially commands whose result is awaiting processing. To await a result before to continue. - Future: .then is a sort of callback. This defines a function invoked when a process is finished.
- Enum. (1.9).
The syntax is very classic, and even somewhat antique, similar to that of JavaScript, created in the 90s and C, created in 1972. This classicism is deliberate. However the language offers very modern features.
Dart vs. TypeScript
Comparison of the syntax of the two candidates to be used in frontend to JavaScript (there are other alternatives).
Dart
void main() {
print("Hello World!");
}
TypeScript
function main() {
console.log("Hello World!");
}
Dart
String str = "Hello in Dart";
TypeScript
var str : string = "Hello in TypeScript";
Dart
var str = "Hello in Dart";
TypeScript
var str = "Hello in TypeScript";
Dart
String x = "$a$b"
TypeScript
var x = a + b;
Dart
if (x == 1) {
print("1");
} else if(x == 2) {
print("2");
} else {
print("x");
}
TypeScript
if (x == 1) {
console.log("1");
} else if(x == 2) {
console.log("2");
} else {
console.log("x");
}
Dart
var x = "x";
switch(x) {
case "a":
print("a");
break;
case "b":
case "c":
print("b or c");
break;
default:
print("x");
}
TypeScript
var x = "x";
switch(x) {
case "a":
console.log("a");
break;
case "b":
case "c":
console.log("b or c");
break;
default:
console.log("x");
}
Dart
var str = "demo";
for(var i = 0; i < str.length; i++) {
print(str[i]);
}
TypeScript
var str = "demo";
for(var i = 0; i < str.length; i++) {
console.log(str[i]);
}
Dart
var arr = [ 1,2,3 ];
for(var x in arr) {
print(x);
}
TypeScript
var arr = [ 1,2,3 ];
for(var x in arr) {
console.log(x)
...
}
Dart
int x;
while(true) {
x++;
}
do {
x++;
} while(true);
TypeScript
var x: number
while(true) {
x++;
}
do {
x++;
} while(true);
Dart
String catstr(String str) {
String x = "Message : $str";
return x;
}
print(catstr("Hello"));
// The type may be omitted.
add(a, b) {
return(a + b);
}
TypeScript
function catstr(str : string) : String {
var x : string = "Message : $str";
return x;
}
console.log(catstr("Hello"));
// The type may be omitted.
function add(a, b) {
return(a + b);
}
Dart
class Vehicle {
int fuel;
int passengers;
Vehicle(int this.speed, int this.passengers) {
}
int distance() {
return(this.fuel / this.passengers);
}
}
class Car extends Vehicle {
int power;
}
TypeScript
class Vehicle {
fuel : number;
passengers : number;
constructor(speed : number, passengers : number) {
}
distance() {
return(this.fuel / this.passengers);
}
}
class Car extends Vehicle {
power : number;
}
The languages are similar and differ mainly by reversing the type and the identifier for TypeScript, a syntax that dates back to the Pascal language! This syntax is used by Google for the Go language that is 2 years older than Dart.
Dart has also other features not in TypeScript, but they are available only on the server-side version. And Dart compiled to JavaScript works only on recent browsers, and not on IE9 for example.
Dart and browsers, WebAssembly
There was a version of Chrome which integrated the Dart virtual machine called Dartium but Microsoft and Apple did not want to adopt this language in their browsers.
Apple is far from wanting to help building web apps. and the community that develops Webkit refused its implementation on the ground that is not part of the standard Web. It's the same thing on the side of Mozilla where one believes that a future version of JavaScript could add the same improvements that brings Dart, mainly the class model and typed variables. We do not mean that Microsoft has issued a negative opinion on the subject, in fact Microsoft chose a different option: to develop TypeScript.
Finally even Google renounced to implement it in Chrome.
According to the authors, Dart will not be compiled to WebAssembly, which is rather a target for static languages such as C or C++. Wasm would have to become the equivalent of the JVM or CLR for this to be considered.
Why use Dart?
The main shortcoming of Dart was tactical and not technical. Positioning itself as an alternative to JavaScript was its mistake. But after the decision (March 2015) to not implement the virtual machine on the browser and instead just compile Dart to JavaScript, this issue is now removed. (Ref).
This language is particularly suited to those familiar with C and C++: the syntax is similar, although somewhat modernized.
It can replace Java on smartphones or be compiled to JS for the browser, but TypeScript is probably better for this second use.
It is used mainly to make applications on Android or iOS with the Flutter framework. It offers the advantage of a same language on any platform.
Over React Native and Node.js on mobiles, its advantage besides speed, is in its development tools.
Exemple of use of Dart for scripting
To begin, download the zip archive from the site, and extract the contents in a directory, for example c:\dart (it only works on Windows).
You can launch the Dart editor and create a project, but then you are faced with a lot of files including a server, that you can't use without having seriously studied the manual.
To start simply we go through the command line instead. In a code editor, type:
main() {
print("Hello World!");
}
Save the script in the hello.dart file in any directory, for example that of dart. The script is launched with the command:
c:\dart\dart-sdk\bin\dart hello.dart
This will display Hello World!
It will be necessary if you want to continue with the language to add its path to the Windows PATH variable, then you have simply to type dart to start a program.
Tools
- Try Dart online. Enter code online to test it. To test the above examples, the code must be placed in a main function, or beside a main function. They have all been verified.
- Flutter. A Dart scripting framework to make fast Android and iOS apps.
Documents and resources
- Dart.dev. Description and specification of the language, and downloads for Windows. A special editor is also available.
- ECMA 408. Standart specification.
- Brendan Eich about Dart. In comments.
See also...
- JavaScript and Harmony. The evolution of the language. The version 6 of ECMAScript brings most of Dart's features.
- How not to create a new programming language. The eternal C syntax is it the best one?