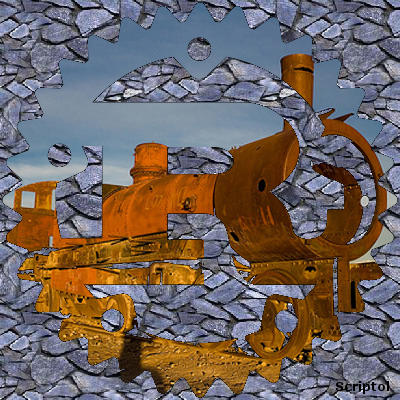
Rust, language of the luddites
Designed by Mozilla to write Servo, potential successor to Firefox, it has an LLVM backend... and a hard coder at the frontend.
The Rust code is available on Github and interests some programmers who see it as a possible successor to C++ in their field.
The word "rust" means red hair in old English and can be an allusion to the fox, symbol of Mozilla. It is also the name of a fungi (think hallucinogenic).
Rust is not a new C to which would have been added dynamic arrays, objects, pattern matching with mixing of different types, automatic memory management, like Vala.
Its main advantage is the safety of memory management, otherwise it takes old principles with new reserved words and syntax, which is probably more expressive, providing we are able to read it. It seems to try to be different for the principle, not for efficiency.
Here is the opinion from the author of "The Cathedral and the Bazaar", Eric Raymond, about Rust:
Even things that should be dirt-simple, like string concatenation, are unreasonably difficult. The language demands a huge amount of fussy, obscure ritual before you can get anything done.
It is clear that the creators of language are not concerned by the gamification of work, and tend rather to the luddite view. It would be preferable for security to be obtained by a more sophisticated backend (see Erlang) than by increased work in coding.
How the compiler works
The steps of generating an executable program are described in the following diagram:
The scanner and the parser produce the AST (Abstract Syntax Tree) code. Optimizations are performed on this code.
Then a semantic analysis is made which performs lots of controls. The 2016 version of the compiler achieves it in two steps: creating an intermediate high level code and then a medium level IR.
The next step is the LLVM code generation, which is made from MIR and in combination with the LLVM API. There is here a choice between producing bitcode, or assembly or object code. In the latter case the LLVM linker produces a binary executable depending on the platform.
Syntax of the language
Comparing the basic elements of Rust with those of C or C++ or a modern language completed by these new elements present in all recent languages.
Rust
fn main() {
println!("Hello World!");
}
C
void main() {
puts("Hello World!");
}
Displaying a string.
Rust
let str = "why not make it complicated";
println!("str: {}", str);
C
char *str = "when it could be simple";
puts(str);
Rust
let alpha: String = "Beta".to_string();
C
char *alpha = "Beta"
In Rust, all local variables are declared by let and are constants! We must add the mut specifier, to be able to reassign them...
Variable in Rust
let a = 1;
a += 1; // Error!
Constant in C
const int a = 1;
a += 1; // Error!
A 'mutable variable' in Rust is a variable (as the name indicates) in C!!!
Mutable variable in Rust
let mut b = 2;
b += 1; // Ok
Variable in C
int b = 2;
b += 1; // Ok
The type is determined by inference, but as it can be ambiguous (and therefore unsafe, it should be noted) the possibility of explicit declaration is added as an option. The coercive nature of the language would have required it the default syntax.
Explicit types in Rust
let b : int = 2;
let ch : char = 'x';
Explicit types in C
int b = 2;
char ch = 'x';
Tuples are mixed lists. An array has the same purpose in other languages.
Tuple in Rust
let t = (4, 5.0, false, "hello");
Mixed array in modern languages
array t = [ 4, 5.0, false, "hello" ]
The switch structure is renamed match to be modern. The bottom line is that it is more elaborate, as in Scala.
Match in Rust
let x : int = 1;
match x {
0 => { println!("Not found"); }
1 => { println!("Found"); }
_ => { println!("Default"); } // default
}
Switch case in C
int x = 1;
switch(x) {
case 0: puts("Not found"); break;
case 1: puts("Found"); break;
default:
println!("Default");
}
The for loop inspired by Python is the only case where Rust is simpler than C. Premiminary versions of the language write: for i in range(0, 10). Then it has been discovered that Scriptol's langage syntax is the best.
Rust
for i in 0..10 {
...
}
C
for(i = 0; i <= 10; i++) {
...
}
Rust uses a special structure for loops with no defined limit, not really necessary.
Rust
let ctr = 0;
loop {
ctr += 1;
if(ctr == 1) {
break;
}
}
C
#define forever 1
int ctr = 0;
while(forever) {
ctr += 1;
if(ctr == 1) {
break;
}
}
The declaration of a function shows the slang aspect of Rust compared to C! Header more complicated with no reason, return simpler but unnecessarily less secure, where is the consistency?
Rust
fn mult(x: int, y: int) -> int {
x * y
}
C
int mult(int x, int y) {
return (x * y);
}
Reading a file line by line.
Rust
let filename = "myfile.txt";
let f = try!(File::open(filename));
let mut file = BufReader::new(&f);
for x in file.lines() {
let line = x.unwrap();
println!("{}", line);
}
C++
char *filename = "myfile.txt";
string line;
ifstream file(filename);
while(getline(file, line)){
cout << line << endl;
}
struct has the role of a classe but methods are added separately, which is an option in C++.
Struct in Rust
struct Point {
x: int,
y: int,
}
impl Point {
fn Start() -> Point {
Point { x: 0, y: 0 }
}
}
Class in C++
class Point {
int x;
int y;
Point Start() {
x = 0;
y = 0;
return this;
}
}
In conclusion, Rust was designed as a system language to replace C++ with a safer memory management, a point we do not deny. Was it necessary to achieve this change by making the syntax so obscure? The motivations of the authors are not clear because the flaws of C, such as semicolons (an error according to the C authors themselves) are retained, but the simplicity of the declarations is not.
The possibilities of the elements of the language were also restricted to reduce the risk of errors. This is something that did not turned well in the past to Pascal or Modula.
Should we use Rust?
Should we use Rust, so choose it rather than Go or C++? We will put Go aside because it competes more with server languages like Java, Python, PHP, the garbage collector may be an annoyance for system programming, and its online modules too, and also the lack of generics.
It remains to choose between the insecurity of C++ when badly used, but with total freedom to achieve what you want, or deal with the obscure syntax of Rust for a safer memory management. They are many reasons for programmers to stay on C++: tons of libraries, all the usefull developement tools, speed of execution...
Reference : Rust reference manual.
Similar projects: Cyclone (ATT), Checked C (Microsoft).